Be the first user to complete this post
|
Add to List |
VBA-Excel: Automate Internet Explorer (IE) using Microsoft Excel – Login into Gmail.
To Automate the Internet Explorer (IE) using Microsoft Excel, say for example you want to login to your gmail account using Microsoft Excel. Please follow the steps mentioned below
Steps:
- Create the object of Internet Explorer
- Make the Internet Explorer visible
- Navigate to the “www.gmail.com”
- Wait till the browser is busy and page is fully loaded
- Get the document Object
- Identify the objects on the Page using “GetElementById”
- Set the Authentication details in the Gmail login page
- Identify the Sign In Button using “GetElementById” and Click on it
Create the object of Internet Explorer
Set objIEBrowser = CreateObject("InternetExplorer.Application")
Make the Internet Explorer visible
objIEBrowser.Visible = True
Navigate to the www.gmail.com
objIEBrowser.Navigate2 "www.gmail.com"
Wait till the browser is busy and page is fully loaded
Do While objIEBrowser.Busy
Loop
Do While objIEBrowser.ReadyState < 4
Loop
You can also call FnWait() for Explicit wait . Click Here
Get the document Object
Set objPage = objIEBrowser.Document
Identify the objects on the Page using “GetElementById”
Set NameEditB = objPage.getElementByID("Email")
Set the Authentication details in the Gmail login page
NameEditB.Value = strUserName
Identify the Sign In Button using “GetElementById” and Click on it
Set SignIn = objPage.getElementByID("signIn")
SignIn.Click
Complete Code:
Function LoginGmail() Set objIEBrowser = CreateObject("InternetExplorer.Application") objIEBrowser.Visible = True objIEBrowser.Navigate2 "www.gmail.com" Call FnWaitForPageLoad(objIEBrowser) Set objPage = objIEBrowser.Document Call FnLogin(objPage, "[email protected]", "MyPassword") Call FnWaitForPageLoad Call FnWait(1) End Function
Function FnLogin(objPage, strUserName, strPwd) Set NameEditB = objPage.getElementByID("Email") Set PWDEditB = objPage.getElementByID("Passwd") NameEditB.Value = strUserName PWDEditB.Value = strPwd Set SignIn = objPage.getElementByID("signIn") SignIn.Click End Function
Function FnWait(intTime) newHour = Hour(Now()) newMinute = Minute(Now()) newSecond = Second(Now()) + intTime waitTime = TimeSerial(newHour, newMinute, newSecond) Application.Wait waitTime End Function
Function FnWaitForPageLoad(objIEBrowser) Do While objIEBrowser.Busy Loop Do While objIEBrowser.ReadyState < 4 Loop End Function
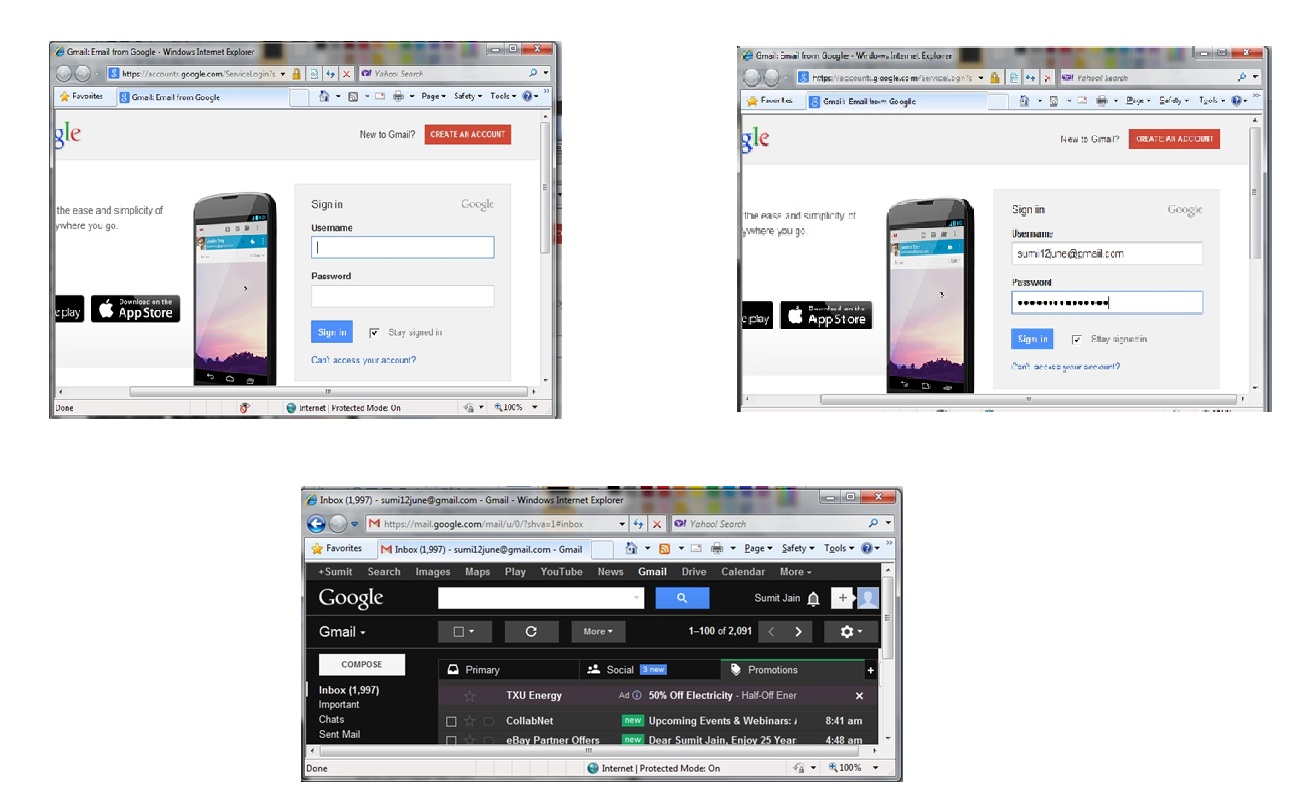