This post is completed by 1 user
|
Add to List |
83. Find Element in Rotated Sorted Array
Objective: Find the given element in the rotated sorted array.
What is a Rotated Sorted Array?
A sorted array is rotated around some pivot element. See the Example Below, the array is rotated after 6.
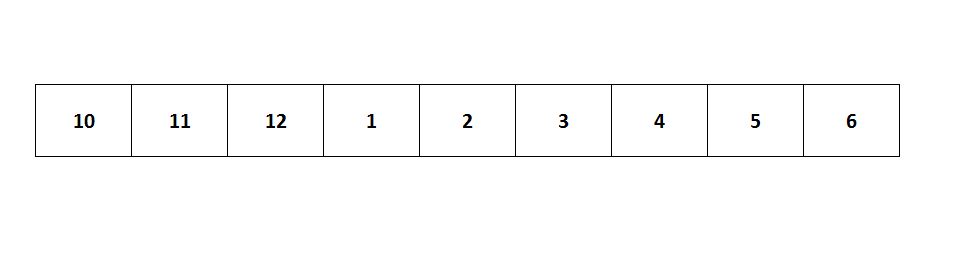
Naive Approach: Do the linear scan and find the element in O(n) time
Better Approach: Binary Search
- Since the array is still sorted we can use binary search to find the element in O(logn) but we cannot apply the normal binary search technique since the array is rotated.
- Say the array is arrA[] and the element that needs to be searched is 'x'.
- Normally when arrA[mid]>arrA[low] we check the left half of the array, and make low = mid-1 but here is the twist, the array might be rotated.
- So first we will check arrA[mid]>arrA[low], if true that means the first half of the array is in strictly increasing order. so next check if arrA[mid] > x && arrA[low] <= x, if true then we can confirm that element x will exist in the first half of the array (so high = mid-1) else array is rotated in the right half and element might be in the right half so (low = mid+1).
- If arrA[mid]>arrA[low], if false that means there is rotation in the first half of the array. so next check if arrA[mid] < x && arrA[high] >= x, if true then we can confirm that element x will exist in the right half of the array (so low = mid+1) else element might be in the first half so (high = mid-1).
Output:
Index of element 5 is 7