Be the first user to complete this post
|
Add to List |
Understanding callbacks in javascript
Ever wonder how callbacks work? Or how you could create your own? Callbacks have been around since a very long time and even though excessive use of callbacks can cause a lot of pain and confusion, callbacks are here to stay. In fact, even code that helps you avoid using callbacks uses callbacks internally(e.g. promises). In this article, I will use simple examples and a visualizations to demonstrate how you can use callbacks so that you can create your own when needed.
Callbacks are nothing but ordinary functions in javascript. This means that you can
- Pass functions as an argument to the other functions, just like you pass variables.
- Return functions from other functions
A Simple Example
Lets take a watered down example of jQuery’s ajax method. Below is the skeleton code of its implementation. [wpgist id="3f349f1d76fcbfc4af5d" file="implementation.js"] In the above example, the second argument to the get function expects a function as the argument. We called itcallback
because it makes it easier to understand, and because its a good convention to have.
Here’s how you would use the get function.
[wpgist id="3f349f1d76fcbfc4af5d" file="usage.js"]
Now lets try to visualize how this works.
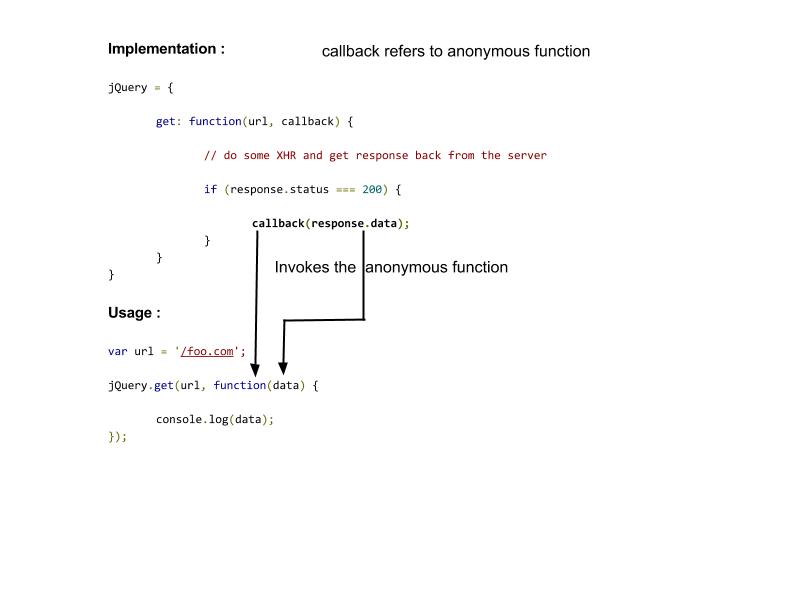
- In the implementation of get function, the callback is actually a reference to the anonymous function passed to it in the usage of get. Why? Because remember we said earlier that a function is just like a variable and can be passed to other functions.
- In the implementation of the get function, when you invoke the callback with response.data, you are invoking that anonymous function with an argument.
Exercise
Perhaps you’d like to learn more about the actual implementation of jQuery’s get function. There’s a nice little source code browser for jQuery by James Padolsey. Take a look at the implementation of the get function there.Also Read:
- Generators and Yield in es6
- Parse html response with fetch API
- position:fixed
- Getting started with automation testing for webrtc applications