Be the first user to complete this post
|
Add to List |
Reactjs flux architecture - Visualized
The react flux architecture example in the TodoMVC repository has quite a bit of code but it takes a long time to understand the relationship between the different parts of the the Flux architecture itself from that code. In an effort to save you (and my future self) time, I put together a visualization of the different pieces of the architecture, the way it is done in the TodoMVC example, and how those parts communicate with each other.
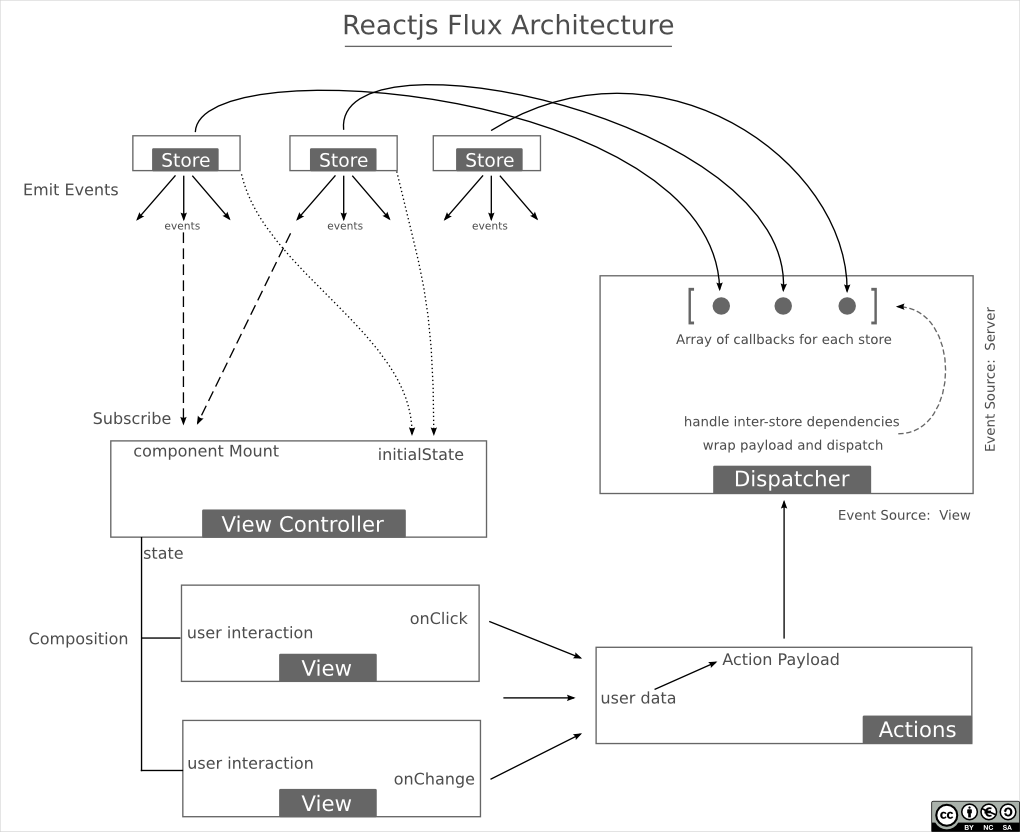
- Stores
- Dispatcher
- View Controllers
- Actions
- Views
Stores
- The only module in which you are allowed to update the value of the underlying model.
- An EventEmitter that emits an appropriate event when the value of the underlying model changes.
- Registers itself with a dispatcher
- When the store registers itself with the dispatcher, it provides a callback method.
- The callback receives at least one argument which is sent from the dispatcher when the callback is invoked. Lets call this object the dispatcher payload.
- In this callback method, the store examines the action attribute of the payload to determine what action needs to be performed on the model/underlying data structure.
- Emits an appropriate event once the model/underlying data structure has changed.
Dispatcher
- Contains a method that allows stores to register themselves with the dispatcher.
- Contains a list of registered stores. Think about this as a store registry.
- Handles payloads from different sources e.g. - Server Side, Client Side (View).
- Takes the payload received from the source.
- Wraps it in an object that allows the handler identify the event source if required. This wrapper object is what I would call the dispatcher payload.
- Invokes each store in the store registry with the dispatcher payload.
View Controller
- Is the master React component/view that contains all other views as sub components of its render method.
- Is the one that subscribes to interesting events on the stores.
- Preferably never initiates change events on the store by itself. This task is left to the sub components/views that actually have the interaction logic.
- Sets the initial state by invoking a method on the store(s) that returns the state of the underlying model.
- Subscribes to store events on component mount.
- Unsubscribes from store events on component unmount.
- In the render method, it passes its own state(which is nothing but the state received from the stores) to the sub components/views so that they can render it as per business logic.
Actions
- Knows about the dispatcher.
- Contains methods that correspond to the actions that the user performs as per business logic. Lets call these methods action methods.
- Action methods receive argument that are nothing but data sent to it from the view that generated the user interacted with.
- The action method can examine the arguments and construct a payload object that contains all the information necessary to perform the requested change in the underlying store.
- The dispatcher is then invoked with this action payload object.
Views
- The only place where user interaction is handled.
- Listens to events on the UI that would lead to a change on the underlying model.
- Delegates the data generated from the user interaction to the appropriate method in the Action(which, as discussed above, will be wrapped into a payload and sent to the dispatcher which is then again re-wrapped and sent to all the registered stores)
Also Read:
- Execution sequence of a React component's lifecycle methods
- Require the css file of a package using webpack
- Using React with Backbone Models
- Render a d3js Tree as a React Component
- webpack with babel6 and react